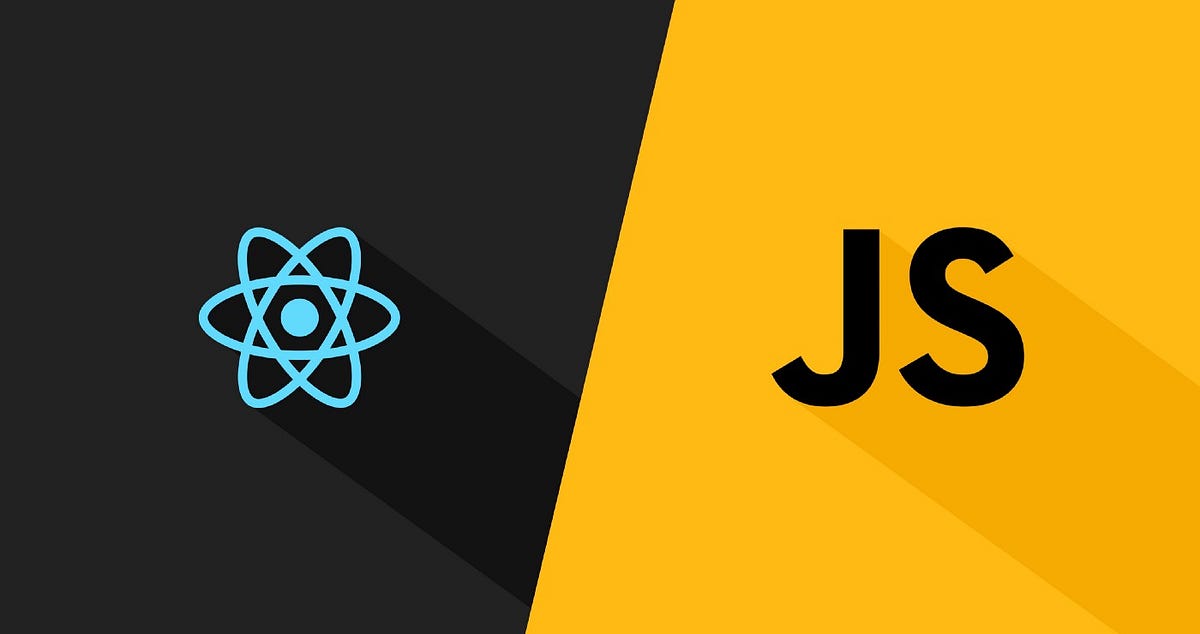
About Course
This course is aimed at learning JavaScript in detail without water, but the main thing is to immediately apply it in practice. This means that you will receive material to work with and together we will create real projects step by step. Theory is good, but without practice there will be no result. Therefore, each theoretical block ends with practice, where you will write an application, interactive elements on the site, etc.
All materials for work will be provided. What is JavaScript and why should you learn it now? JS is the language for interactivity on web pages. Nowadays not a single website on the Internet can do without it. And even more! Using various frameworks, JavaScript is sweeping the internet: server side (Node.js), mobile apps (React Native, Ionic), virtual reality (React 360) and so on. Therefore, if you want to go down one of these paths, native JS will be an essential base for you.
What Will You Learn?
- Learn the basics of programming and algorithms
- Learn basic JavaScript concepts and principles, from the simplest to the most complex.
- Learn popular technologies such as AJAX, JSON, etc.
- Learn to work with Git and GitHub
- Learn to work with npm, Babel, Browserify, Webpack, Heroku, Firebase, etc.
- Find out which framework or library to choose in the future. Get to know React, Angular, Vue, Jquery
- Explore the React library and absolutely everything connected with it (including Redux)
- Learn to create full-fledged web applications
- Reinforce everything you learned on real projects
Course Content
Preparing for work
-
How to take this course
-
Why this course?
-
Symbols and materials
-
Setting up your workspace
-
About code editors and tutorials
-
(e) Eslint. Error hints and automatic code formatting
JavaScript Basics
-
What is JS and how to connect it to a page
-
About exercises and additional lessons
-
Variables and strict mode
-
Rules and types of variable naming
-
Creating the Correct Variables Challenge
-
Classification of data types in JavaScript
-
The difference between objects and arrays and non-obvious syntactic possibilities
-
The challenge of creating the right data types
-
Simple communication with the user
-
Interpolation (ES6)
-
Operators in JS
-
Learning to work with the Git version control system and the GitHub service
-
How to work with GitHub from different computers, gitignore and Git Kraken
-
Network protocols. Connecting your computer to your Github account via SSH
-
Practice, part 1. Let’s start creating the application
-
Conditions
-
Logical operators
-
Cycles
-
Loop within a loop and labels
-
Tasks on using cycles and conditions
-
Advanced tasks using loops and conditions
-
Figure formation task
-
Practice, part 2. Applying conditions and loops
-
Functions, arrow functions (ES6)
-
Once again about function arguments
-
About the importance of return
-
Task for working with functions
-
Trim() method
-
Callback functions
-
Objects, object destructuring (ES6)
-
Arrays and pseudo-arrays
-
Algorithms in general and in JavaScript
-
Pass by reference or by value, Spread operator (ES6-ES9)
-
Tasks for working with objects
-
Tasks for working with arrays
-
Tasks for working with arrays, part 2
-
OOP Basics, Prototype-Based Inheritance
-
Practice, part 4. Using objects
-
Advanced task for working with objects and arrays
-
Advanced task for working with objects and arrays
-
We catch errors in our code using the developer console. Breakpoints
-
Dynamic typing in JS
-
Closure and Lexical Environment
-
Intermediate test to consolidate knowledge
-
Navigation through DOM elements, data attributes, for/of advantage
-
Recursion
-
Recursion problem
-
Resources to sharpen your programming skills
Additional JavaScript Basics
-
About additional information on the basics
-
Null Merge Operator (Nullish, ??) ES11
-
Optional chain operator (?.) ES11
-
Living Collections and Helpful Techniques
-
Symbol data type
-
Property Descriptors and Useful Object Methods
-
Iterable Constructs
-
Map
-
Set
-
BigInt
-
Complex problem of calculating the number of pages
-
A difficult task involving working with strings
-
Difficult task to work with recursion
JavaScript in action
-
What will this module be about
-
ClassList and event delegation
-
Creating tabs in a new project
-
Scripts and their execution time. setTimeout and setInterval
-
(e) Garbage collector and memory leaks
-
(*) WeakMap and WeakSet
-
Working with dates
-
Create a countdown timer on the website
-
(*) Processing past date
-
Constructor functions
-
Call context. This
-
Classes (ES6)
-
Where to track information about new standards
-
Using classes in real work
-
Rest operator and default parameters (ES6)
-
Module knowledge consolidation test
Advanced JavaScript
-
Local servers
-
JSON data transfer format, deep cloning of objects
-
AJAX and communication with the server
-
Implementation of a script for sending data to the server
-
Beautiful user notification
-
Promise (ES6)
-
Fetch API
-
Array Iteration Methods
-
Tasks for working with array methods
-
Tasks for working with array methods
-
Details about npm and the project. JSON-server
-
Getting data from the server. Async/Await (ES8)
-
Additionally: What are libraries. axios library
-
Creating a slider on the website, option 1
-
Creating a slider on the site, option 2
-
Getters and setters (object properties)
-
Encapsulation
-
Reception module, how and why to use it
-
Webpack. Putting our project together
-
ES6 Modules
-
Putting our project together and fixing bugs
-
Building a portfolio on GitHub
-
Errors. How to avoid breaking your code
-
Making your own mistakes
-
How to convert ES6+ code to legacy ES5 format. Babel, Core.js and polyfills
-
Modern libraries and frameworks
-
Jquery library
-
Macro and microtasks
-
We work with ready-made code
-
Final practical-theoretical test
-
What projects can you come up with and implement yourself